At Torq, we use gRPC as our one and only synchronous communication protocol. Microservices communicate with each other using gRPC, our external API is exposed via gRPC and our frontend application (written using VueJS) uses the gRPC protocol to communicate with our backend services. One of the main strengths of gRPC is the community and the language support. Given some proto files, you can generate a server and a client for most programming languages. In this article, I will explain how to communicate with your gRPC backend using the great gRPC-web OSS project.
A quick overview of our architecture
We are using a microservice architecture at Torq. When we initially started, each microservice had an external and internal API endpoint.
After working that way for several months, we realized it doesn’t work as well as we’d imagined. As a result, we decided to adopt the API Gateway/Backend For Frontend approach.
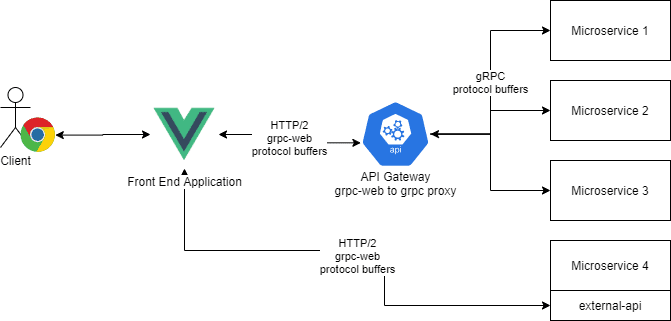
gRPC-web is a JavaScript implementation of gRPC for browser clients. It gives you all the advantages of working with gRPC, such as efficient serialization, a simple IDL, and easy interface updating. A gRPC-web client connects to gRPC services via a special proxy, as shown below.

Envoy has built in support for this type of proxy.
Here you can also find an example of the grpc-web proxy implementation in Golang. We use a similar implementation at Torq.
Building gRPC Web clients
We generate gRPC clients for all our external APIs during the CI process. In the case of gRPC-web client, an npm package is generated and then published to the GitHub package repository. Then, JavaScript applications can consume this package using the npm install
command.
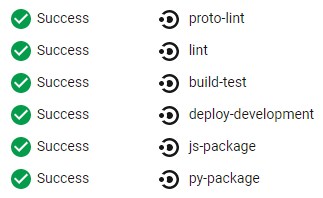
Sample client/server example
Our proto interface
This is a really simple service. You send it GetCurrentTimeRequest
and it returns GetCurrentTimeResponse
containing the text representation of time.Now().String()
.
Generating clients and servers
In order to generate the clients and the servers for this proto file you need to use the protoc command. Generating gRPC-web client and JavaScript definitions requires the protoc-gen-grpc-web plugin. You can get it here or use the pre-baked docker image jfbrandhorst/grpc-web-generators that contains all the tools needed to work with grpc-web.
This is the command I’m using to generate both the Go clients/servers and the JavaScript clients:
It will put the Go clients in ./time/goclient
and the JavaScript clients in ./frontend/src/jsclient
.
It’s worth noting that the client generator is also able to generate TypeScript code, which you can read more about in its documentation.
Backend
A really basic Go server which just listens on 0.0.0.0:8080
. It implements the TimeServiceServer interface
and returns time.Now().String()
for each request.
Frontend
Using gRPC-web in the frontend is pretty simple, as shown by the example below.
A small tip – I recommend enabling the gRPC-web chrome extension. It’s a great way to inspect your gRPC traffic coming from and to the browser, just like you would with the Network Activity Inspector that is built into Chrome.
Envoy configuration
Like I previously mentioned, gRPC-web needs a proxy to translate into gRPC. Envoy has native support for this, and the following configuration example for Envoy does exactly that.
Final words
I hope this article will help you easily dive into gRPC-web. It is a great technology, especially if you are already using gRPC everywhere. We’re using it with great success in our frontend application. If you’re interested in learning more, you can get started with the source code for this article here.